LynxJS is an exciting new technology just introduced from ByteDance, the team behind TikTok.
This is Part 1 of a 2 part series looking at native platform handling comparisons between LynxJS and NativeScript.
- → Part 1: Native Module Creation
- Part 2: Reuse existing native platform code
LynxJS has provided documentation on Native Module creation here.
When developing Lynx applications, you may encounter scenarios where you need to interact with native platform APIs not covered by Lynx. Or, you might want to reuse existing native platform code in your Lynx application. Regardless of the reason, you can use Native Modules to seamlessly connect your JavaScript code with native code, allowing you to call native platform functions and APIs from your JavaScript code. The following will detail how to write a native module.
Let's compare the process outlined in this document to how you can do the same with NativeScript.
If you're looking for a comparison on reusing existing native platform code, see Part 2 here.
Comparison in 1 Snapshot
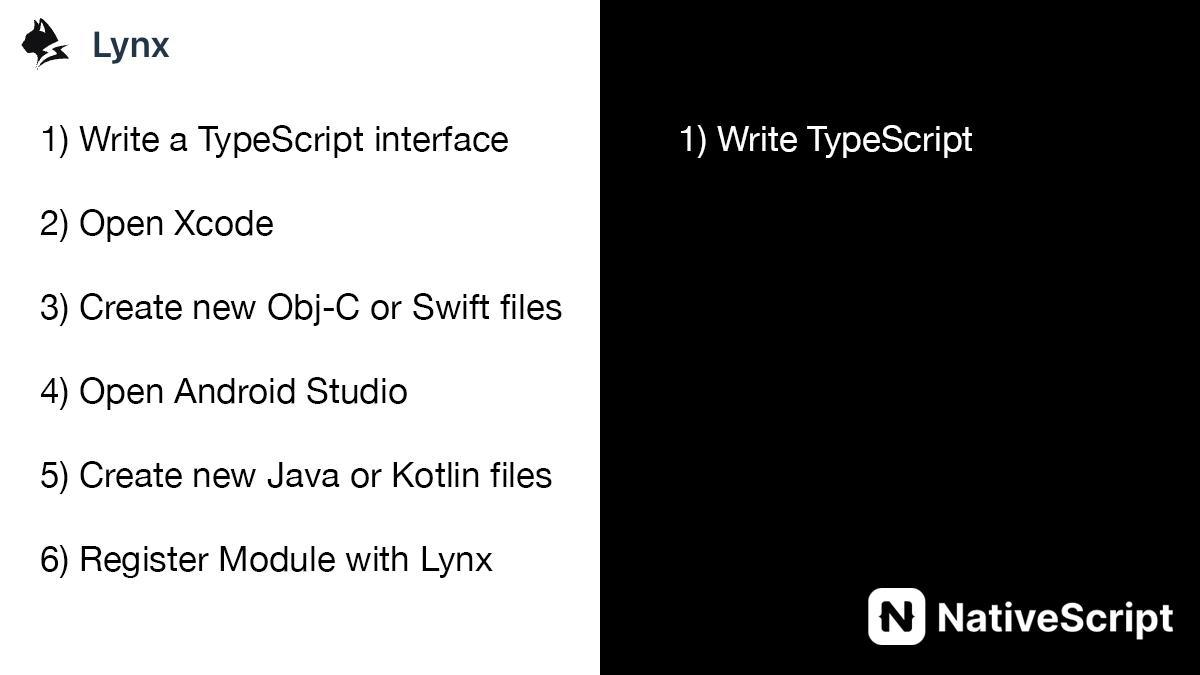
Create a Native Module with LynxJS
You can follow this documentation: https://lynxjs.org/guide/use-native-modules#platform=ios
Create a Native Module with NativeScript
Note: You would never need to write this example since @nativescript/core already provides a simple unified API via ApplicationSettings.
But let's pretend we need to do this.
We can just write TypeScript.
import { Utils } from '@nativescript/core';
const deviceStorage = () => {
if (__APPLE__) {
return NSUserDefaults.standardUserDefaults;
} else {
return Utils.android.getApplicationContext().getSharedPreferences('MyLocalStorage', 0);
}
};
const setStorageItem = (key: string, value: string) => {
if (__APPLE__) {
deviceStorage().setObjectForKey(value, key);
} else {
const editor = deviceStorage().edit();
editor.putString(key, value);
editor.apply();
}
};
const getStorageItem = (key: string) => {
if (__APPLE__) {
return deviceStorage().stringForKey(key);
} else {
return deviceStorage().getString(key, '');
}
};
const clearStorage = () => {
if (__APPLE__) {
deviceStorage().removePersistentDomainForName(NSBundle.mainBundle.bundleIdentifier);
} else {
deviceStorage().edit().clear().apply();
}
}
Use the TypeScript you just wrote.
No Xcode, no Obj-C, no Swift, no Android Studio, no Kotlin, no Java, no Registering a module.
Try on StackBlitz right now:
- With Angular: https://stackblitz.com/edit/nativescript-native-api-comparison-ng?file=src%2Fapp%2Fitem%2Fitems.component.ts
- With React: https://stackblitz.com/edit/nativescript-native-api-comparison-react?file=src%2Fcomponents%2FScreenOne.tsx
- With Solid: https://stackblitz.com/edit/nativescript-native-api-comparison-solid?file=src%2Fcomponents%2Fhome.tsx
- With Svelte: https://stackblitz.com/edit/nativescript-native-api-comparison-svelte?file=app%2Fcomponents%2FHome.svelte
- With Vue 3: https://stackblitz.com/edit/nativescript-native-api-comparison-vue?file=src%2Fcomponents%2FHome.vue
Additional Notes
NativeScript provides direct access to all native APIs as well as cross-platform capabilities through @nativescript/core, third party plugins or @nativescript/* plugins. Additionally, you can easily add custom native source files directly to your project and use them immediately from TypeScript, even still without having to jump through hoops as directed in LynxJS docs. See this documentation which goes further to describe how to interop with platform source files if ever needed.
Takeaways
LynxJS is really neat, check it out! Documentation: https://lynxjs.org/guide/start/quick-start.html
What would make it better? 🤔 To be able to use NativeScript with it.